HOWTO: Use the Zetafax API in .NET
Print
ZTN1476
ID: ZTN1476
This Zetafax technical note applies to:
Summary
The Zetafax API allows third-party applications to submit faxes to the Zetafax Server for sending, and to control and monitor their progress.
The COM API can be easily used for development in a .NET environment. This document provides some simple example code to illustrate how the Zetafax API can be used in .NET
More information
Registering the ZfAPI.dll
Like all COM components ZfAPI32.dll must be registered before it can be used. This can be done by following the technote HOWTO: Develop and distribute your fax-enabled application.
Adding the COM object to a .NET project
To use the COM API in a .NET project, the COM object should be referenced as follows:
(The images shown here are taken from Microsoft Visual Studio .NET)
- Select the "Add Reference" option from the Solution Explorer pane.
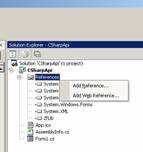
- A dialog will open with a list of COM components to select from. Scroll down and select the "Zfapi32 1.x Type library" (in the image below the version selected is 1.4). Once selected the entry will be been added to the list of "Selected Components" (The listbox at the bottom of the dialog). Press the "OK" button to dismiss the dialog.
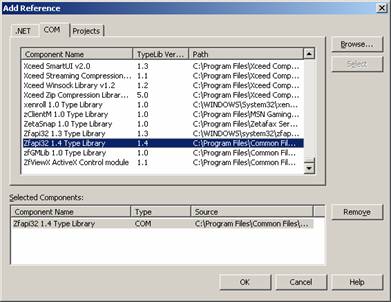
A reference has now been created to the COM API object.
Code Samples
The following samples are written in C# and Visual Basic .NET. The other .NET languages (Managed C++, Visual J#) are very similar and these examples can easily be adapted for use in these environments.
Note: To avoid the usage of long type-names declare the following namespace at the top of the file:
using ZfLib;
The API fully supports exception handling and it is recommended that you use the try-catch mechanism to receive meaningful error messages and descriptions from the Zetafax COM API . (The examples below demonstrate this).
- The following example demonstrates sending a fax using the COM API:
VB.NET:
' Declare objects
Dim oZfAPI As New ZfLib.ZfAPI
Dim oUserSession As ZfLib.UserSession
Dim oNewMessage As ZfLib.NewMessage
Try
' Logon and create NewMessage:
oUserSession = oZfAPI.Logon(" ADMINIST" , False)
oNewMessage = oUserSession.CreateNewMsg
' Set properties:
oNewMessage.Recipients.AddFaxRecipient(" Sam Smith" , _
" ACME plc" , _
" 020 7123 4567" )
oNewMessage.Text = " I am a fax!"
oNewMessage.Priority = ZfLib.PriorityEnum.zfPriorityUrgent
' Send!
oNewMessage.Send()
Catch ex As Exception
System.Windows.Forms.MessageBox.Show(ex.Message,
" Zetafax Error" ,
MessageBoxButtons.OK,
MessageBoxIcon.Error)
End Try
C#:
// Declare objects
ZfAPIClass oZfAPI = new ZfAPIClass();
UserSession oUserSession;
ZfLib.NewMessage oNewMessage;
try
{
// Logon and create NewMessage:
oUserSession = oZfAPI.Logon(" ADMINIST" , false);
oNewMessage = oUserSession.CreateNewMsg();
// Set properties:
oNewMessage.Recipients.AddFaxRecipient(" Sam Smith" , //TO
" ACME plc" , //Organization
" 020 7123 4567" );//Fax number
oNewMessage.Text = " I am a fax!" ;
oNewMessage.Priority = ZfLib.PriorityEnum.zfPriorityUrgent;
// Send!
oNewMessage.Send();
}
catch (System.Runtime.InteropServices.COMException ex)
{
System.Windows.Forms.MessageBox.Show(ex.Message,
" Zetafax Error" , MessageBoxButtons.OK, MessageBoxIcon.Error);
}
2. The code below demonstrates message information handling:
VB.NET:
' Declare objects:
Dim strBody As String
Dim oZfAPI As New ZfLib.ZfAPI
Dim oUserSession As ZfLib.UserSession
Dim oMessage As ZfLib.Message
Dim oMsgHist As ZfLib.MessageHistory
Try
strBody = " ~ZAPI001"
' Logon and get message:
oUserSession = oZfAPI.Logon(" ADMINIST" , False)
oMessage = oUserSession.Outbox.GetMsg(strBody)
' Display information about the message
With oMessage.GetMsgInfo()
txtCaption.Text = .Body
txtSubject.Text = .Subject
txtComment.Text = .Comment
End With
' Display the number and call duration for each recipient:
Dim MessageHistoryEnum As IEnumerator
MessageHistoryEnum = oMessage.GetMsgHistories.GetEnumerator()
While MessageHistoryEnum.MoveNext
oMsgHist = MessageHistoryEnum.Current
lstHistory.Items.Add(" To: " & oMsgHist.Name & _
" Time Taken:" & oMsgHist.Date.ToString())
End While
Catch ex As Exception
System.Windows.Forms.MessageBox.Show(ex.Message,
" Zetafax Error" , MessageBoxButtons.OK, MessageBoxIcon.Error)
End Try
C#:
// Declare objects:
string strBody;
ZfAPIClass oZfAPI = new ZfAPIClass();
UserSession oUserSession;
ZfLib.Message oMessage;
ZfLib.MessageHistory oMsgHist;
try
{
strBody = " ~ZAPI001" ;
// Logon and get message:
oUserSession = oZfAPI.Logon(" ADMINIST" , false);
oMessage = oUserSession.Outbox.GetMsg(strBody);
// Display information about the message
txtCaption.Text = oMessage.GetMsgInfo().Body;
txtSubject.Text = oMessage.GetMsgInfo().Subject;
txtComment.Text = oMessage.GetMsgInfo().Comment;
// Display the number and call duration for each recipient:
string szFormattedHistoryItem;
IEnumerator MessageHistoryEnum = oMessage.GetMsgHistories().GetEnumerator( );
while (MessageHistoryEnum.MoveNext())
{
oMsgHist = (ZfLib.MessageHistory) MessageHistoryEnum.Current;
szFormattedHistoryItem = string.Format(" To:{0} TimeTaken:{1}" ,
oMsgHist.Name,
oMsgHist.Date.ToString());
lstHistory.Items.Add(szFormattedHistoryItem);
}
}
catch (System.Runtime.InteropServices.COMException ex)
{
System.Windows.Forms.MessageBox.Show(ex.Message,
" Zetafax Error" , MessageBoxButtons.OK, MessageBoxIcon.Error);
}
3. The following code demonstrates how to retrieve Zetafax device information:
VB.NET:
' Declare objects:
Dim oZfAPI As New ZfLib.ZfAPI
Dim oUserSession As ZfLib.UserSession
Dim oDevices As ZfLib.Devices
Dim oDevice As ZfLib.Device
Try
' Logon and get devices:
oUserSession = oZfAPI.Logon(" ADMINIST" , False)
oDevices = oUserSession.Server.GetServerInfo().Devices
' Enumerate devices adding information to Listbox:
Dim DeviceEnum As IEnumerator
DeviceEnum = oDevices.GetEnumerator()
While DeviceEnum.MoveNext
'Iterate through the devices
oDevice = DeviceEnum.Current
lstDevices.Items.Add(oDevice.Name & " " & oDevice.User)
End While
Catch ex As Exception
System.Windows.Forms.MessageBox.Show(ex.Message,
" Zetafax Error" , MessageBoxButtons.OK,
MessageBoxIcon.Error)
End Try
C#:
// Declare objects:
ZfAPIClass oZfAPI = new ZfAPIClass();
UserSession oUserSession;
ZfLib.Devices oDevices;
ZfLib.Device oDevice;
try
{
// Logon and get devices:
oUserSession = oZfAPI.Logon(" ADMINIST" , false);
oDevices = oUserSession.Server.GetServerInfo().Devices;
// Enumerate devices adding information to Listbox:
IEnumerator DeviceEnum = oDevices.GetEnumerator();
while(DeviceEnum.MoveNext())
{
oDevice = (ZfLib.Device)DeviceEnum.Current;
lstDevices.Items.Add(oDevice.Name + " " + oDevice.User);
}
}
catch (System.Runtime.InteropServices.COMException ex)
{
System.Windows.Forms.MessageBox.Show(ex.Message,
" Zetafax Error" ,
MessageBoxButtons.OK,
MessageBoxIcon.Error);
}
References
HOWTO: Develop and distribute your fax-enabled application
For more information on using the Zetafax COM API please refer to the Zetafax API compiled help.
MSDN - .NET Framework Developer Centre on Microsoft .NET Framework on MSDN
Last reviewed: 11 November 2005 (GC/EB)
Date Published: 27 June 2005 (AS/DH)